Decentralized Blockchain with OrbitDB, IPFS, P2P & Express.js
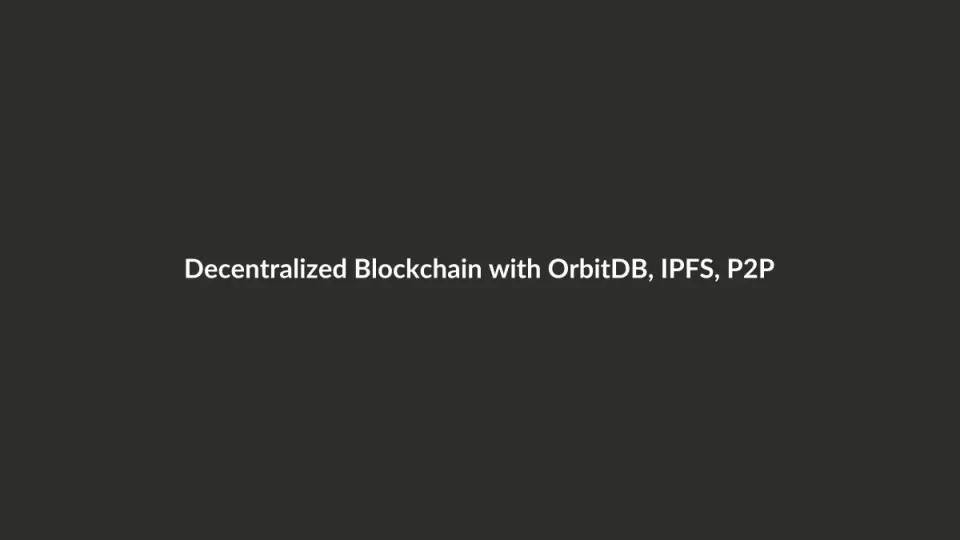
🚀 Decentralized Blockchain with OrbitDB, IPFS, P2P & Express.js
This is a TypeScript-based blockchain system using OrbitDB, IPFS, cryptography, P2P networking, and Express.js. It includes:
✅ Block mining
✅ Transactions
✅ Wallet balances
✅ REST API for interaction
✅ P2P network communication
1️⃣ Blockchain Core Implementation
📌 Block Structure
Each block contains:
- Index (position in the chain)
- Timestamp (when mined)
- Transactions (list of transfers)
- Previous hash (link to the last block)
- Nonce (for Proof-of-Work)
- Hash (block identifier)
block.ts
import crypto from "crypto";
import { Transaction } from "./transaction";
export class Block {
constructor(
public index: number,
public timestamp: number,
public transactions: Transaction[],
public previousHash: string = "",
public nonce: number = 0
) {
this.hash = this.calculateHash();
}
hash: string;
calculateHash(): string {
return crypto
.createHash("sha256")
.update(
this.index +
this.timestamp +
JSON.stringify(this.transactions) +
this.previousHash +
this.nonce
)
.digest("hex");
}
mineBlock(difficulty: number): void {
while (this.hash.substring(0, difficulty) !== "0".repeat(difficulty)) {
this.nonce++;
this.hash = this.calculateHash();
}
console.log(`⛏️ Block mined: ${this.hash}`);
}
}
2️⃣ Blockchain Structure & Mining
📌 The blockchain stores:
- Blocks
- Verifies transactions
- Allows mining new blocks
blockchain.ts
import { Block } from "./block";
import { Transaction } from "./transaction";
import { P2PNode } from "./p2p";
export class Blockchain {
private chain: Block[];
private transactionPool: Transaction[];
private p2pNode: P2PNode;
private difficulty: number = 3;
private miningReward: number = 50;
constructor(p2pNode: P2PNode) {
this.p2pNode = p2pNode;
this.chain = [this.createGenesisBlock()];
this.transactionPool = [];
}
createGenesisBlock(): Block {
return new Block(0, Date.now(), [], "0");
}
getLatestBlock(): Block {
return this.chain[this.chain.length - 1];
}
addTransaction(transaction: Transaction): boolean {
this.transactionPool.push(transaction);
return true;
}
minePendingTransactions(minerAddress: string): void {
const block = new Block(
this.chain.length,
Date.now(),
this.transactionPool,
this.getLatestBlock().hash
);
block.mineBlock(this.difficulty);
this.chain.push(block);
this.transactionPool = [new Transaction("System", minerAddress, this.miningReward)];
}
isValidChain(): boolean {
for (let i = 1; i < this.chain.length; i++) {
const currentBlock = this.chain[i];
const previousBlock = this.chain[i - 1];
if (currentBlock.hash !== currentBlock.calculateHash()) return false;
if (currentBlock.previousHash !== previousBlock.hash) return false;
}
return true;
}
}
3️⃣ Transactions & Wallets
Transactions include:
- Sender (wallet address)
- Recipient (destination address)
- Amount (value being transferred)
- Signature (for authentication)
transaction.ts
import crypto from "crypto";
export class Transaction {
constructor(
public sender: string,
public recipient: string,
public amount: number,
public signature?: string
) {}
signTransaction(privateKey: string): void {
const signer = crypto.createSign("sha256");
signer.update(this.sender + this.recipient + this.amount);
this.signature = signer.sign(privateKey, "hex");
}
}
4️⃣ P2P Network with WebSockets
This enables node-to-node communication, syncing the blockchain across multiple machines.
p2p.ts
import WebSocket from "ws";
export class P2PNode {
private sockets: WebSocket[] = [];
constructor(private port: number) {}
startServer(): void {
const server = new WebSocket.Server({ port: this.port });
server.on("connection", (ws) => {
this.sockets.push(ws);
ws.on("message", (message) => {
console.log("📡 Received:", message.toString());
});
});
console.log(`🌍 P2P Node running on port ${this.port}`);
}
connectToPeer(peer: string): void {
const ws = new WebSocket(peer);
ws.on("open", () => this.sockets.push(ws));
}
broadcast(message: string): void {
this.sockets.forEach((socket) => socket.send(message));
}
}
5️⃣ REST API with Express.js
Allows external applications to interact with the blockchain.
server.ts
import express from "express";
import { Blockchain } from "./blockchain";
import { Transaction } from "./transaction";
import { P2PNode } from "./p2p";
const app = express();
app.use(express.json());
const p2pNode = new P2PNode(6001);
const blockchain = new Blockchain(p2pNode);
app.get("/blocks", (req, res) => {
res.json(blockchain);
});
app.post("/transaction", (req, res) => {
const { sender, recipient, amount, privateKey } = req.body;
const transaction = new Transaction(sender, recipient, amount);
transaction.signTransaction(privateKey);
blockchain.addTransaction(transaction);
res.json({ message: "Transaction added" });
});
app.post("/mine", (req, res) => {
const { minerAddress } = req.body;
blockchain.minePendingTransactions(minerAddress);
res.json({ message: "Mining completed" });
});
app.listen(5000, () => console.log("🚀 API running on port 5000"));
6️⃣ Wallet Balance API
Calculates a wallet’s balance based on past transactions.
server.ts
(updated)
app.get("/balance/:address", async (req, res) => {
const address = req.params.address;
if (!address) return res.status(400).json({ message: "Wallet address is required" });
let balance = 0;
for (const block of blockchain.chain) {
for (const transaction of block.transactions) {
if (transaction.sender === address) balance -= transaction.amount;
if (transaction.recipient === address) balance += transaction.amount;
}
}
res.json({ address, balance });
});
7️⃣ How to Run
1. Install Dependencies
npm install express ws crypto
2. Run the Server
tsx server.ts
3. Interact with the API
✅ View Blockchain
curl -X GET http://localhost:5000/blocks
✅ Create Transaction
curl -X POST http://localhost:5000/transaction -H "Content-Type: application/json" -d '{
"sender": "Alice",
"recipient": "Bob",
"amount": 10,
"privateKey": "Alice_Private_Key"
}'
✅ Mine Transactions
curl -X POST http://localhost:5000/mine -d '{
"minerAddress": "Miner_Wallet"
}'
✅ Check Balance
curl -X GET http://localhost:5000/balance/Alice
✅ Conclusion
This blockchain system provides decentralization, transactions, mining, wallet balances, and P2P syncing using OrbitDB, IPFS, libp2p, and cryptography. 🚀
🔮 Future Enhancements
To make this blockchain more robust and production-ready, consider the following improvements:
1️⃣ Security Enhancements
- Prevent Double Spending: Implement UTXO (Unspent Transaction Output) model or transaction validation before adding to the blockchain.
- Better Hashing & Encryption: Use elliptic curve cryptography (ECC) for key generation and transaction signing.
- Consensus Algorithm Upgrade: Instead of PoW, explore PoS (Proof of Stake) or DPoS (Delegated PoS) for faster transactions.
2️⃣ Full P2P Network Sync
- Implement Gossip Protocol for efficient node discovery.
- Use libp2p’s pub-sub mechanism to broadcast new transactions and blocks.
- Ensure chain reorganization in case of conflicting forks.
3️⃣ Smart Contracts & DApps
- Introduce WASM-based smart contracts for on-chain business logic.
- Develop a simple VM (Virtual Machine) or script execution engine.
- Enable off-chain computations for complex operations.
4️⃣ IPFS & OrbitDB Integration
- Store block headers & transaction references in IPFS for decentralization.
- Use OrbitDB for an immutable transaction ledger instead of in-memory storage.
5️⃣ Scaling & Performance
- Implement Sharding & Layer 2 solutions (e.g., State Channels, Rollups) for scalability.
- Optimize block size & mining difficulty dynamically.
6️⃣ API & Frontend for Real-World Use
- Develop a web dashboard & wallet for managing transactions.
- Implement GraphQL API for querying blockchain data efficiently.
- Create a mobile app for seamless user interaction.
🚀 Final Thoughts
This blockchain serves as a foundation for building decentralized applications, offering a secure and scalable P2P network.
By integrating OrbitDB, IPFS, and libp2p, it achieves true decentralization and off-chain storage.
The next step is to optimize, scale, and deploy it in real-world applications—whether for DeFi, supply chain tracking, secure messaging, or IoT device management.
🛠 Keep innovating, keep decentralizing! 🚀💡